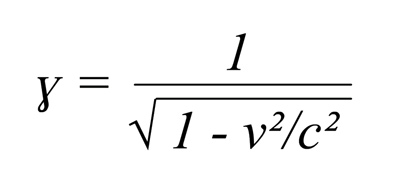
Relative Gamma
Time Dilation Due to Relative Speed Difference Speed: Standing StillRunning: 7mphBicycle: 20mphCar: 60mphNascar: 200mphTypical Jet: 575mphSpeed of Sound: 768mphSpin of the Earth …
Time Dilation Due to Relative Speed Difference Speed: Standing StillRunning: 7mphBicycle: 20mphCar: 60mphNascar: 200mphTypical Jet: 575mphSpeed of Sound: 768mphSpin of the Earth …
00_introduction Introduction to Python¶ This guide is strictly about Python3. It is specifically for use with Python versions: 3.6, 3.7 and 3.8. …
01_hello_world Simple Python I/O¶ The most common I/O functions in Python are input() and print(). These are not the only I/O functions …
02_numbers Python Numbers¶ Python has several built-in types for general programming. It also supports custom types known as Classes. Not all classes …
03_text Python Strings¶ Strings are very powerful in Python. No other language has a string quite like Python. String Formatting¶ There are …
04_ordered_array Python Sequences Are Ordered Arrays¶ Python Sequences come in two flavors – list and tuple. List: sequential array (fexible). my_list = …
05_membership_array Python Sets Are Membership Arrays¶ Sets are homogeneous collections of unique values. A literal set is defined like a list – …
06_associative_array Dictionary: Ordered Associative Array¶ Dictionaries are also known as hashtables. The keys of a dictionary must be hashable. The values may …
07_control_flow Control Flow¶ There are three basic types of control flow operation; Branch, Iteration and Jump. The most powerful of the three …
08_callable_objects Callable Object: Functor¶ In Python the most common type of functor is the Function. There are many other types of functors …
09_modules Modules & Packages¶ Python files are modules. A folder of Python files (with one special file added: __init__.py) is a package. …
10_class_objects Classes¶ Classes are the object factories of many programming languages. The objects that classes create are typically called instances. Classes can …
11_deep_copy Deep vs Shallow Copy¶ Facts and Myths about Python | YouTube.com Ned Batchelder In [0]: from copy import deepcopy In [0]: a = …
12_parameter_packs Parameter Packs¶ Star Args: *some_sequence Star Star Key Wargs: **some_dictionary Keyword Only Arguments Star Args¶ Let’s say we have a function …
13_iterators Iterators & Iterables¶ All iterators are iterable, but not all iterables are iterators. Iterators have state. They remember where they left …
14_generators Generators¶ Generators are custom iterators. Generator Function Generator Expression Reinventing the Parser Generator | YouTube.com David Beazley Generators are one way …
15_decorators Decorators¶ Function Decorator Class Decorator Decorators allow us to modify existing classes or functions without redefining them. Practical decorators | YouTube.com …
16_advanced_classes Advanced Class Topics¶ Python’s Class Development Toolkit | YouTube.com Raymond Hettinger Super Function¶ The super function is required when more than …
17_deployment Module Installation & Deployment¶ PyPi is also known as the cheese shop. This is the Python Package Index, a public repository …
18_extensions Basic Cython Extension¶ Cython | cython.org First we need to make sure Cython is installed.¶ $ pip install Cython … Create …
19_custom_tooling Custom Tooling¶ High-performance Polyhedral Dice Function Timer File: Dice.hpp¶ C++ header-only library #pragma once #include <random> #include <algorithm> namespace Dice { …